In the Python Course #8 you learned everything about Python list
, list
slicing, and all the list
functions that Python provides. In this article, you will learn about another sequential data type, the tuple
. The data type tuple
is inspired by the tuples in mathematics (Wikipedia) and in stark contrast to Python list
s tuple
s are an immutable data type.
Free Python Tuples Cheat Sheet
Pick up your free Python tuples cheat sheet from my Gumroad shop:
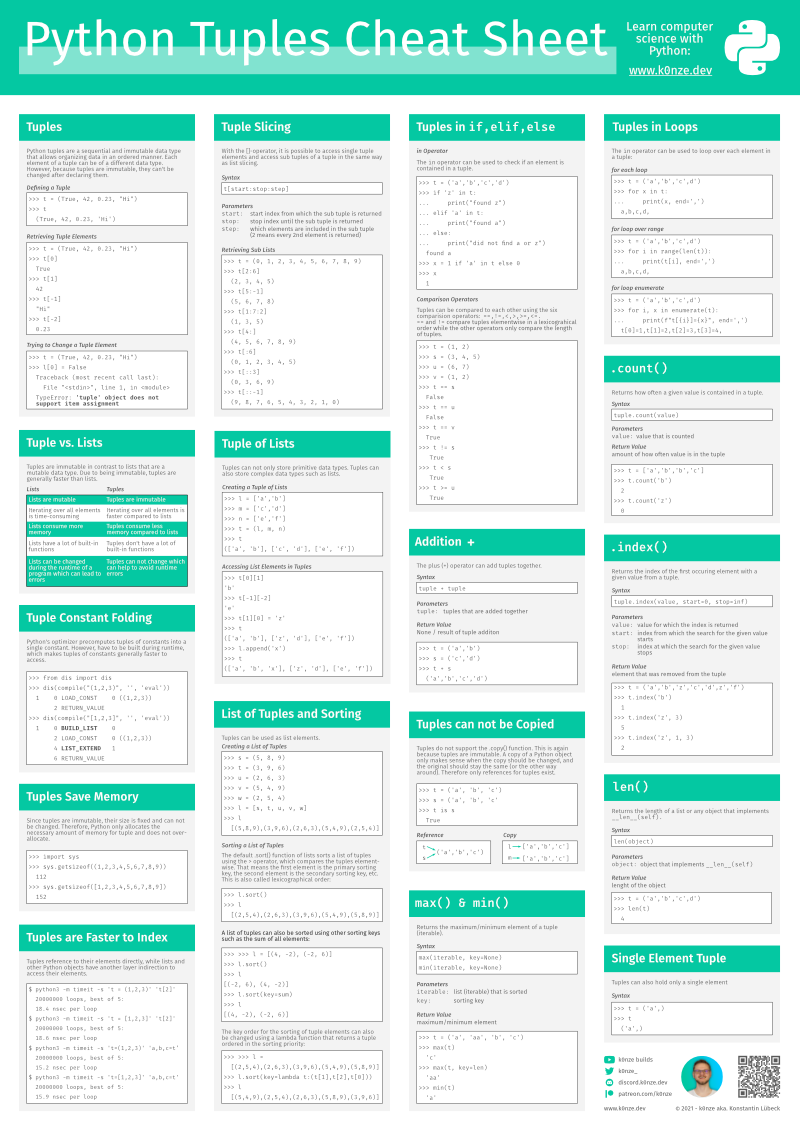
Declaring a Python Tuple
To declare a new tuple
in Python, all the elements that should be included in the tuple
are written in between parenthesis (
)
separated by commas:
1
2
3
>>> t = (True, 42, 0.23, "Hi")
>>> t
(True, 42, 0.23, 'Hi')
Accessing Python Tuple Elements
To retrieve the elements from a tuple
, the []
-operator is used with the same indexing schema as in Python list
s where the first element is an index 0
and the last element can be accessed with index -1
:
1
2
3
4
5
6
7
8
9
>>> t = (True, 42, 0.23, "Hi")
>>> t[0]
True
>>> t[1]
42
>>> t[-1]
'Hi'
>>> t[-2]
0.23
Python Tuple Slicing
The []
-operator also allows slicing in the same way as with list
(Python Course #8 on list
slicing):
1
2
3
4
5
6
7
8
9
10
11
>>> i = (1, 2, 3, 4, 5, 6, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
>>> i[4:14]
(5, 6, 6, 7, 8, 9, 10, 11, 12, 13)
>>> i[7:]
(7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
>>> i[:5]
(1, 2, 3, 4, 5)
>>> i[3:17:4]
(4, 7, 11, 15)
>>> i[::3]
(1, 4, 6, 9, 12, 15, 18)
Changing Python Tuple Elements
Unlike Python list
s tuple elements can’t be changed because tuple
s are an immutable data type. And if you try to change a tuple
element using the []
-operator, you are presented with a TypeError
:
1
2
3
4
5
>>> t = (True, 42, 0.23, "Hi")
>>> t[0] = False
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
Adding Python Tuples Together with +
Even though you can’t change tuple
s, you can add tuple
s together, or to be extra fancy concatenate them, to create a new tuple
using +
1
2
3
4
>>> t = ('a','b')
>>> s = ('c','d')
>>> t + s
('a', 'b', 'c', 'd')
Python Immutable Data Types (are faster)
You might ask yourself now, what is a tuple
suitable for if you can’t change its values? Python tuple
s are generally “faster” than lists, so accessing all elements in a list
takes longer than accessing all elements in a tuple
. The following examples use the Python package timeit
to measure the run time of a piece of code. In the first example, all elements of a list
are combined into a string using the join
operation. This is done 1000000 times to magnify the effect (see the parameter number
). In the second part a tuple
containing the same elements such as the list
is used for the join
operation and executed 1000000 as well:
1
2
3
4
5
6
7
import timeit
list_time = timeit.timeit('"".join(["a","b","c","d","e","f","g","h","i","j","k","l","m"])', number=1000000)
print("list: ", list_time)
tuple_time = timeit.timeit('"".join(("a","b","c","d","e","f","g","h","i","j","k","l","m"))', number=1000000)
print("tuple:", tuple_time)
The result of this comparison should look like this where the join
over tuple
s is roughly 1.5 times faster than a join
over a list (your numbers can vary depending on your computer hardware):
1
2
list: 0.16607940000000002
tuple: 0.10540459999999999
List of Python Tuples
This performance comparison might look like an artificial example; however if you got a big data set and want to perform operations on it, a speedup of 1.5 could be pretty significant. Such a data set could be coordinates used for route planning in a navigation system for autonomous driving and could be stored in a list
:
1
2
3
>>> l = [(0.25,0.13), (0.51,0.86), (0.92,0.12), (0.64,0.72)]
>>> l
[(0.25, 0.13), (0.51, 0.86), (0.92, 0.12), (0.64, 0.72)]
Tuple of Python Lists
tuple
s can not only store primitive data types such as bool
, int
, float
, or str
. tuple
s can contain any data type you want. For example you can declare a tuple
that contains list
s:
1
2
3
4
5
6
>>> l = ['a','b']
>>> m = ['c','d']
>>> n = ['e','f']
>>> t = (l, m, n)
>>> t
(['a', 'b'], ['c', 'd'], ['e', 'f'])
To access an element of a list
contained in t
you can stack the []
-operator:
1
2
3
4
>>> t[0][1]
'b'
>>> t[-1][-2]
'e'
By stacking the []
-operator you can also change the elements contained in the list
s:
1
2
3
>>> t[1][0] = 'z'
>>> t
(['a', 'b'], ['z', 'd'], ['e', 'f'])
But how is that possible if tuple
s are immutable? When declaring a tuple
a reference to the list
is stored in the tuple; that reference is immutable while the list
is still mutable. You can also still change the list
outside of the tuple
:
1
2
3
>>> l.append('x')
>>> t
(['a', 'b', 'x'], ['z', 'd'], ['e', 'f'])
This distinction between a reference and a value is crucial for understanding Python, and you will see it a lot during this Python course.
Python Sorting a List of Tuples
When tuple
s are stored in a list
you can use the list
.sort()
function. The default behavior of the .sort()
function uses the >
comparison operator. It sorts the tuples by its elements while the first tuple
element is the primary key, the second element is the secondary key, etc.
1
2
3
4
>>> l = [(5,8,9), (3,9,6), (2,6,3), (5,4,9), (2,5,4)]
>>> l.sort()
>>> l
[(2,5,4),(2,6,3),(3,9,6),(5,4,9),(5,8,9)]
It is also possible to use a different sorting key such as the sum of all tuples elements:
1
2
3
4
5
6
7
>>> l = [(4, -2), (-2, 6)]
>>> l.sort()
>>> l
[(-2, 6), (4, -2)]
>>> l.sort(key=sum)
>>> l
[(4, -2), (-2, 6)]
Python Tuples with a Single Element
Even though it is not very useful, you can declare a tuple
only containing one element. The declaration of such a one-tuple
looks a bit weird because there is a comma added after the first element that is not followed by a second element:
1
2
>>> t = ('a',)
>>> t
Python Tuple Functions
Such as Python list
s tuple
s also come with functions that allow you to access certain properties of a tuple
.
tuple()
A list
can be turned into a tuple
using the tuple()
function and vice versa a tuple can be turned into a list with the list
function:
1
2
3
4
5
6
7
>>> l = ['a','b','c']
>>> t = tuple(l)
>>> t
('a', 'b', 'c')
>>> m = list(t)
>>> m
['a', 'b', 'c']
len()
To get the length of a tuple
use the len()
function:
1
2
3
>>> t = ('a','b','c')
>>> len(t)
3
Comparing Python Tuples
tuple
s can also be compared to each other using the comparison operations: ==
, !=
, >
, <
, >=
, <=
. The operators compare the tuple
s elementwise in the lexicograhical order.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
>>> t = (1,2)
>>> s = (3,4,5)
>>> u = (1,2)
>>> t == s
False
>>> t == u
True
>>> t != s
True
>>> t > s
False
>>> t < s
True
>>> t >= u
True
>>> t <= s
True
in
If you want to check if an element is contained in a tuple use the in
operator:
1
2
3
4
5
>>> t = ('a','b','c')
>>> 'a' in t
True
>>> 'z' in t
False
min() / max()
Getting the minimum or maximum element of a tuple
can be achieved with the min()
/max()
function:
1
2
3
4
5
>>> t = (1,2,3,4)
>>> min(t)
1
>>> max(t)
4
.index()
To get the index of a value contained in a tuple
use the .index()
function:
1
2
3
4
5
6
7
>>> t = ('a','b','c')
>>> t.index('a')
0
>>> t.index('z')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: tuple.index(x): x not in tuple
.count()
To check how often an element is included in a tuple
use .count()
:
1
2
3
4
5
6
7
>>> t = ('a','b','b','c')
>>> t.count('a')
1
>>> t.count('b')
2
>>> t.count('z')
0
As the Python tuple
is an immutable data type, it doesn’t offer a lot of functions because it can not be changed after its declaration.
The .count()
function concludes this article on Python tuple
s. Make sure to get the free Python Tuples Cheat Sheet in my Gumroad shop. If you have any questions about this article, feel free to join our Discord community to ask them over there.